Scripting Documentation: Difference between revisions
No edit summary |
No edit summary |
||
(5 intermediate revisions by the same user not shown) | |||
Line 13: | Line 13: | ||
Here we will cover the basic steps on creating a new object usable within OpenClonk. | Here we will cover the basic steps on creating a new object usable within OpenClonk. | ||
Because creating each new object from scratch would be tedious, repetitive work, we provide a template object to work with | Because creating each new object from scratch would be tedious, repetitive work, we provide a template object to work with. | ||
'''Step 1''' | '''Step 1''' | ||
Line 25: | Line 25: | ||
[DefCore] | [DefCore] | ||
id=TemplateObject | id=TemplateObject | ||
Version= | Version=5,2,0,1 | ||
Category=C4D_Object | Category=C4D_Object | ||
Width=8 | Width=8 | ||
Line 40: | Line 40: | ||
</pre> | </pre> | ||
DefCores contain a fair amount of basic object data, but for now we only need to change the ID to something unique; for example: | |||
<pre width=40> | <pre width=40> | ||
[DefCore] | [DefCore] | ||
id=MyObject | id=MyObject | ||
Version= | Version=5,2,0,1 | ||
...etc. | ...etc. | ||
</pre> | </pre> | ||
Line 153: | Line 89: | ||
====Make it Bounce!==== | ====Make it Bounce!==== | ||
For this example, we'll make | For this example, we'll make use of the variables given by the Hit callback. The Hit callback is a function called by the engine whenever an object strikes the ground with sufficient force. | ||
The Hit function used in the previous example didn't make use of the variables which the Hit callback uses, so we'll have to add those into the script. Formerly the Hit function was written as such: | |||
<pre width=40> | |||
protected func Hit() | protected func Hit() | ||
{ | { | ||
// | |||
} | } | ||
</pre> | </pre> | ||
Within the brackets, add "int xdir, int ydir". This will allow the Hit callback to receive the x speed and y speed of the object when it hit the ground. Now we can use these variables within the Hit function and manipulate the object's speed to simulate a bounce. | |||
To do this, a simple reversal of the ydir is enough. To set an object's y speed, we use the function "SetYDir()". The new speed we wish the object to have will be written inside the brackets (the first parameter). Of course, to reverse a value, all we have to do is multiply the number by negative one. There is another thing we need to pay attention to specific to the Hit function; the variables for xdir and ydir have a precision value of 100. Therefore, in the SetYDir function we must define that (the second parameter). | |||
The script should be written something like this: | |||
<pre width=50> | |||
protected func Hit(int xdir, int ydir) | |||
{ | |||
SetYDir(ydir * -1, 100); | |||
return 1; | |||
}</pre> |
Latest revision as of 20:22, 5 October 2011
C4Script
C4Script is the C-like script-language used by OpenClonk. C4Script controls in-game objects; from the Clonk to the GUI, everything is programmed with C4Script.
The C4Script Reference Library is an online resource containing documentation of nearly all C4Script functions, as well as information on syntax and how to use many different features of the language.
As a side note, the name 'C4Script' comes from the first game it was used in, Clonk 4. Each following game in the series has vastly expanded on and improved the language since it's inception.
An Introduction to C4Script
Learning a new script language can be difficult, especially if you do not have any previous programming knowledge. This introduction will appeal to these users, as we do not expect any new developer to have a firm basis in C before starting. ;-)
Creating your First Object
Here we will cover the basic steps on creating a new object usable within OpenClonk.
Because creating each new object from scratch would be tedious, repetitive work, we provide a template object to work with.
Step 1
Copy the template into the root directory of OpenClonk (the folder containing your game executable), and rename it to something such as 'MyObject.ocd'. Now have a look inside your object's folder. There are a few things we'll need to change before it will load as your object, instead of over-riding the template.
The DefCore
Open the DefCore.txt file. You'll see something similar to this:
[DefCore] id=TemplateObject Version=5,2,0,1 Category=C4D_Object Width=8 Height=8 Offset=-4,-4 Vertices=3 VertexX=0,2,-2 VertexY=1,-1,-1 VertexFriction=20,20,20 Value=1 Mass=10 Components=Rock=1;Wood=1; Rotate=1
DefCores contain a fair amount of basic object data, but for now we only need to change the ID to something unique; for example:
[DefCore] id=MyObject Version=5,2,0,1 ...etc.
Everything else in the DefCore is fine for our purposes, so let's have a look at the script.
The Script File
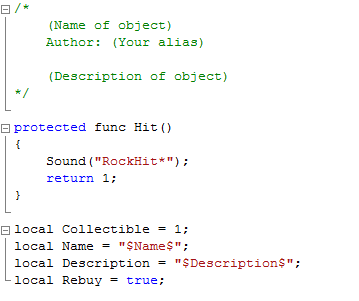
The script file contains the object's 'code', or the 'programming' of the object. This is the real meat of custom objects.
If you use Windows, to edit Script files in a more user-friendly way it is suggested you use a program such as Notepad++ instead of the default text editor.
Have a look at the 'Hit()' function. Currently when the object hits the landscape, it makes a RockHit* sound. Let's make it explode instead. ;D
Delete the line containing the Sound function, and write 'Explode(30);' instead:
protected func Hit() { Explode(30); return 1; }
Script Tip: Notice every line ends with a semi-colon. If a line is not closed, the engine will throw a syntax error! The same goes for brackets, ' { } or ( ) '. |
Also, make sure to modify the header for you object. Add the name, a description, and fill in the author! :]
Test your object!
Now it's time to try out your object in game. Run the game, and start a test scenario. Hit Ctrl+F5 to start Dev-mode, and type this in as a message (replace 'MyObject' with the ID of your object):
/script GetCursor()->CreateContents(MyObject) |
Your clonk should have the new object appear in his inventory. Throw it on the ground!
Other Fun Example Scripts for your Object
These examples will make you familiar with a few common script functions.
Make it Bounce!
For this example, we'll make use of the variables given by the Hit callback. The Hit callback is a function called by the engine whenever an object strikes the ground with sufficient force.
The Hit function used in the previous example didn't make use of the variables which the Hit callback uses, so we'll have to add those into the script. Formerly the Hit function was written as such:
protected func Hit() { // }
Within the brackets, add "int xdir, int ydir". This will allow the Hit callback to receive the x speed and y speed of the object when it hit the ground. Now we can use these variables within the Hit function and manipulate the object's speed to simulate a bounce.
To do this, a simple reversal of the ydir is enough. To set an object's y speed, we use the function "SetYDir()". The new speed we wish the object to have will be written inside the brackets (the first parameter). Of course, to reverse a value, all we have to do is multiply the number by negative one. There is another thing we need to pay attention to specific to the Hit function; the variables for xdir and ydir have a precision value of 100. Therefore, in the SetYDir function we must define that (the second parameter).
The script should be written something like this:
protected func Hit(int xdir, int ydir) { SetYDir(ydir * -1, 100); return 1; }