Tutorial: Map Generator - Algorithms & Transformations
This is the second part of map generator tutorial. The first part can be found here, and should be completed before trying this tutorial. Here two aspects of the map generator will be explained: algorithms and transformations. Both are attributes to the objects map and overlay, although using them in overlays makes more sense.
[TODO: Explain algorithms] Explain algorithms, they can be found here in the map generator documentation. [TODO: Explain transformations]
Alternative checkers board
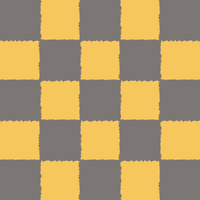
We ended the previous part with an exercise about logical operators and set theory. The landscape shown in the figure had to be constructed, we will now see that there are alternative methods to construct such a landscape. Such an alternative is to use an algorithm. We need the checker
algorithm, which creates an overlay consisting of alternating boxes of size ten. The problem we then still have is that we have a full checkers board, which we want to reduce to half its size. For this purpose we can use the attributes zoomX
and zoomY
to zoom out by a factor of two. All together results in the following code.
// Checker algorithm at work. map ExampleChecker { mat=Gold; tex=gold; overlay { mat=Rock; tex=rock; algo=checker; zoomX=50; zoomY=50; }; };
Convince yourself that this produces the same landscape as in the exercise. We encounter here a major advantage of algorithms, they reduce the amount of overlays needed drastically.
Bozo Algorithm
Let's introduce another algorithm, therefore consider the following piece of map generator code.
// Golden specks. map ExampleBozo { mat=Rock; tex=rock; overlay { mat=Gold; tex=gold; algo=bozo; a=2; rotate=45; }; };
[TODO: explanation of bozo and rotate and image here]
Landscape with hills
It is time to produce a first real landscape, we want to create a landscape with two hills separated by a lake.
// Two hills separated by a lake. map ExampleHills { // First raise the water to a certain level. overlay { mat=Water; tex=water; x=0; y=50; wdt=100; hgt=50; }; // Then overlay two hills using the sine algorithm. overlay { mat=Earth; tex=earth; algo=sin; zoomX=60; zoomY=35; ox=25; oy=35; }; };
[TODO: Explanation here too]