Tutorial: Creating A Parkour
This tutorial provides a basis for scenario designers wanting to create a parkour. It explains the basics and some extended features of the parkour goal. Furthermore some elementary design issues for parkours will be addressed.
Parkour
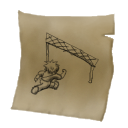
A parkour is basically an extended race, players have to get from start to finish but have to pass checkpoints in between. The parkour goal gives the scenario designer options to adjust the path, thereby increasing the challenge for the players. Other features would be creating team parkours, where team members need to split up and complete different paths to join forces later. On the player's side rounds get less frustrating since they would only have to redo parts of a parkour after having died, as you respawn at the latest passed checkpoint.
Scenario script
The parkour goal can not be created through a Scenario.txt entry, since the checkpoints have to be created manually. Thus the obvious location to create the parkour goal is in the scenario script, an example of such a script is given here.
/*-- Parkour --*/
protected func Initialize()
{
// First create the goal and store it.
var goal = CreateObject(Goal_Parkour);
// Place the start at the left of the map.
goal->SetStartpoint(50, LandscapeHeight() / 2);
// Place some checkpoints from left to right at random height in between.
var cp_count = 10;
for (var cp_num = 1; cp_num <= cp_count; cp_num++)
{
var x = cp_num * LandscapeWidth() / (cp_count + 1);
var y = Random(LandscapeHeight());
var cp_mode = PARKOUR_CP_Check | PARKOUR_CP_Respawn | PARKOUR_CP_Ordered;
goal->AddCheckpoint(x, y, cp_mode);
}
// Place the finish at the right of the map.
goal->SetFinishPoint(LandscapeWidth() - 50, LandscapeHeight() / 2);
return;
}
// Callback from parkour goal to the scenario script.
protected func PlrHasRespawned(int plr, object cp)
{
// Give the player a shovel when he respawns.
var clonk = GetCrew(plr);
clonk->CreateContents(Shovel);
}
This of course cries for an explanation. First note that for a static map, i.e. those created from a Map.bmp, you can look for decent places for your checkpoints and create them. For dynamic maps you need to let a script search a decent location, in this example script we do not worry about such issues. So in the first line the parkour goal is created and stored in the variable goal, cause we need that later. The second line creates the parkour start, only create one start checkpoint. The function
public func SetStartpoint(int x, int y)
creates a start checkpoint at the global coordinates x and y and returns the checkpoint just created. After that 10 checkpoints are created ordered along the x direction at random height, for this the function
public func AddCheckpoint(int x, int y, int mode)
is used. This creates a checkpoint at the global coordinates x and y with the specified mode and returns the checkpoint just created. Checkpoint modes will be explained later, just note here that the checkpoints created in the example have to be passed in order and respawn the player at the latest passed checkpoint. In the last line the the parkour finish is created, also here only one finish should be created. The function
public func SetFinishpoint(int x, int y)
creates a finish checkpoint at the global coordinates x and y and returns the checkpoint just created. The parkour goal also provides a callback to the scenario script
OnPlayerRespawn(int plr, object cp)
when the player is (re)spawned at a checkpoint. The player number and the checkpoint are passed as parameters. With this callback you can give the player necessary equipment to overcome the challenges provided.
Checkpoints
Checkpoint Modes
A checkpoint can have various modes, these can be assigned using a bit mask. The different modes are
- PARKOUR_CP_Start: Marks the start of a parkour, players spawn at this checkpoint. This mode is not compatible with other modes, and only one can be placed.
- PARKOUR_CP_Finish: Marks the finish of a parkour. This mode is compatible with PARKOUR_CP_Team and only one can be placed.
- PARKOUR_CP_Check: This mode has to be set for the basic checkpoint functionality, meaning that a player must have passed this in order to be able to finish the parkour.
- PARKOUR_CP_Respawn: If this mode is set, the checkpoint will respawn players. The checkpoint at which will be respawned is the last checkpoint of this mode passed by the player or the start.
- PARKOUR_CP_Ordered: This checkpoint has to be completed in logic order. If you have multiple ordered checkpoints, an ordered checkpoint can only be completed if the previous ordered checkpoint has been passed.
- PARKOUR_CP_Team: Normally only one team member needs to pass a checkpoint. With this mode all team members must have passed this checkpoint.
Parkour Design
Landscape
When designing scenario's for clonk the designer has the choice between static and dynamic landscapes. This section will elaborate a bit on the influence of the landscape on a parkour.
- Static landscape: The major disadvantage of having a static landscape is the loss of replayability. Unlike melees other player's choice do not affect your strategy much, they could throw stuff at you and alter the landscape but that's about it. Therefore after having played several rounds on a static map the fun starts to drop. Static maps however do provide a better base for riddle-like cooperative parkours, since it is easier to provide specific challenges to the player.
- Dynamic landscape: The advantage of a dynamic map over a static one is the replayability. Dynamic maps don't have drop in replay value after a few rounds, cause every map provides a unique challenge. A disadvantage is that dynamic maps are harder to create, and checkpoint placement is also more difficult.