Tutorial: Map Generator - Randomness
This is the third part of the map generator tutorial. The previous part can be found here, and should be completed before trying this part of the tutorial. In the two previous parts you learned how to create overlays and combined them with logical operators, use algorithms and how to transform them. These ingredients do allow you to build some basic maps already, as seen in the previous exercise, but we need one more aspect of the map generator to make landscapes really interesting. That aspect is randomness and is treated in this part of the tutorial. There are two ways randomness is introduced into maps, these are through algorithms that behave randomly and through a single transformation called turbulence.
Turbulent Lines

So let's start with introducing turbulence. For this purpose a new algorithm is introduced, the lines algorithm. It draws straight vertical lines where the parameter a
determines the line width and b
the distance between the lines. Therefore b
must always be chosen larger than a
, otherwise the whole overlay will be filled completely. Furthermore we will introduce two new attributes which together specify the turbulence transformation. First there is the turbulence
attribute - which takes the values 10, 100, 1000 and 10000 - specifying the amount of deformation of the layer. Second the lambda
attribute can be used to repeat the deformation process up to 20 times. So now let's take a look at this piece of map generator code and the result.
// Straight lines made interesting. map ExampleTurbulentLines { mat=Water; tex=water; overlay { algo=lines; a=10; b=20; mat=Gold; tex=gold; turbulence=10; lambda=0; }; };
You will have noticed that the straight golden lines of the algorithm are deformed quite smoothly by the applied turbulence. Turbulence uses a sine function to shift randomly shift the points of a layer, which transforms regular shapes into disordered and curved ones. Now try larger values for the turbulence
and lambda
attributes and observe there effects. Turbulence is a crucial attribute and you will need it in almost any dynamic map you design.
Random Points
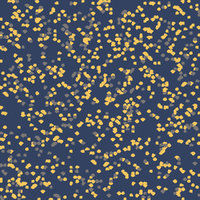
Random behaviour in the map generator can also be induced through the use of algorithms, the random algorithm is one of these. It draws random points, the parameter a
determines the likelihood a point is being drawn. A point is less likely drawn if a
is increased.
// Random gold and rock points. map ExampleRandomPoints { mat=Water; tex=water; overlay { algo=random; a=10; mat=Gold; tex=gold; }; overlay { algo=random; a=30; mat=Rock; tex=rock; }; };
In this example we see that quite a few golden points are drawn in the water. On top of this some rock points are drawn. As expected a lot less rock points were drawn, cause a
is higher in that overlay. Note that zoom transformations do not have any effect on the point size of this algorithm. As a side effect the other transformations won't have an effect either because points are the smallest quantity the map generator works on.
Random Checker
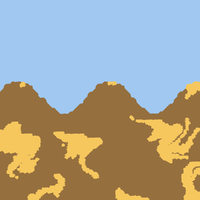
Let's consider the exercise in the previous part, where a simple landscape suitable for mining gold had to be created. The gold was put into the earth using the bozo algorithm, resulting in a pretty generic landscape. For a more interesting landscape it is vital to have in-earth resources like gold at random locations. This can be achieved by using the rndchecker algorithm, which behaves like the checker algortihm but places the boxes randomly. However there is still the problem that all specks look identical, for bozo all are ellipses and for rndchecker all are squares. Therefore we ought to use turbulence and zoom to transform the boxes into larger and randomly deformed specks.
map ExampleRandomChecker { // Use the sine algorithm for some small hills. overlay { mat=Earth; tex=earth; algo=sin; zoomX=20; zoomY=-20; ox=20; oy=40; // Use the rndchecker algorithm for some gold in the earth. overlay { mat=Gold; tex=gold; algo=rndchecker; a=3; zoomX=10; zoomY=-35; turbulence=100; lambda=3; }; }; };
So we took the map generator code from the previous exercise and replaced the bozo algorithm with rndchecker algorithm. The parameter a
determines the chance a box is placed, where higher a
means a lower chance. Zoom was used to stretch the golden specks in the horizontal direction a little, and some turbulence was applied to obtain random shapes. So we observe that the rndchecker algorithm combined with zoom and turbulence provides a nice tool to create in-earth resources.
Exercise: Sky Islands
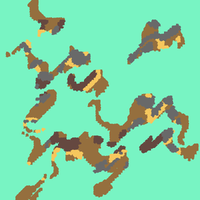
In this exercise you must create a landscape suitable for settlement, consisting of randomly placed sky islands. To keep things simple the sky islands must consist of earth filled with gold, coal and ore. Create something looking similar to the landscape in the figure.
[TODO: solution as some sort of spoiler]
// Sky islands filled with resources. map ExerciseSkyIslands { // Use the rndchecker algorithm to create sky islands. overlay { mat=Earth; tex=earth; x=10; wdt=80; y=10; hgt=80; algo=rndchecker; a=1; zoomX=20; zoomY=-10; turbulence=100; lambda=2; loosebounds=1; // Use the rndchecker algorithm for some gold, coal and ore in the earth. overlay { mat=Gold; tex=gold; algo=rndchecker; a=3; zoomX=10; zoomY=-35; turbulence=100; lambda=3; }; overlay { mat=Coal; tex=coal; algo=rndchecker; a=4; zoomX=10; zoomY=-35; turbulence=100; lambda=3; }; overlay { mat=Ore; tex=ore; algo=rndchecker; a=5; zoomX=10; zoomY=-35; turbulence=100; lambda=3; }; }; };
Summary
So you learned how to apply turbulence and to use random algorithms. Together with the things learned in part one and two, this allows you to create complete landscapes. However there are still some interesting special features of the map generator that need to be discussed, this is done in the next part.